01_GriddedDiagnostics_TempAdvection_CFSR: Compute derived quantities using MetPy
Contents
01_GriddedDiagnostics_TempAdvection_CFSR: Compute derived quantities using MetPy¶
In this notebook, we’ll cover the following:¶
Select a date and access various CFSR Datasets
Subset the desired Datasets along their dimensions
Calculate and visualize diagnostic quantities.
Smooth the diagnostic field.
0) Preliminaries ¶
import xarray as xr
import pandas as pd
import numpy as np
from datetime import datetime as dt
from metpy.units import units
import metpy.calc as mpcalc
import cartopy.crs as ccrs
import cartopy.feature as cfeature
import matplotlib.pyplot as plt
ERROR 1: PROJ: proj_create_from_database: Open of /knight/anaconda_aug22/envs/aug22_env/share/proj failed
1) Specify a starting and ending date/time, and access several CFSR Datasets¶
startYear = 2007
startMonth = 2
startDay = 14
startHour = 12
startMinute = 0
startDateTime = dt(startYear,startMonth,startDay, startHour, startMinute)
endYear = 2007
endMonth = 2
endDay = 14
endHour = 12
endMinute = 0
endDateTime = dt(endYear,endMonth,endDay, endHour, endMinute)
Create Xarray Dataset
objects¶
dsZ = xr.open_dataset ('/cfsr/data/%s/g.%s.0p5.anl.nc' % (startYear, startYear))
dsT = xr.open_dataset ('/cfsr/data/%s/t.%s.0p5.anl.nc' % (startYear, startYear))
dsU = xr.open_dataset ('/cfsr/data/%s/u.%s.0p5.anl.nc' % (startYear, startYear))
dsV = xr.open_dataset ('/cfsr/data/%s/v.%s.0p5.anl.nc' % (startYear, startYear))
dsW = xr.open_dataset ('/cfsr/data/%s/w.%s.0p5.anl.nc' % (startYear, startYear))
dsQ = xr.open_dataset ('/cfsr/data/%s/q.%s.0p5.anl.nc' % (startYear, startYear))
dsSLP = xr.open_dataset ('/cfsr/data/%s/pmsl.%s.0p5.anl.nc' % (startYear, startYear))
2) Specify a date/time range, and subset the desired Dataset
s along their dimensions.¶
Create a list of date and times based on what we specified for the initial and final times, using Pandas’ date_range function
dateList = pd.date_range(startDateTime, endDateTime,freq="6H")
dateList
DatetimeIndex(['2007-02-14 12:00:00'], dtype='datetime64[ns]', freq='6H')
# Areal extent
lonW = -90
lonE = -60
latS = 35
latN = 50
cLat, cLon = (latS + latN)/2, (lonW + lonE)/2
latRange = np.arange(latS,latN+.5,.5) # expand the data range a bit beyond the plot range
lonRange = np.arange(lonW,lonE+.5,.5) # Need to match longitude values to those of the coordinate variable
Specify the pressure level.
# Vertical level specificaton
pLevel = 850
levStr = f'{pLevel}'
We will display temperature and wind (and ultimately, temperature advection), so pick the relevant variables.
Now create objects for our desired DataArrays based on the coordinates we have subsetted.¶
# Data variable selection
T = dsT['t'].sel(time=dateList,lev=pLevel,lat=latRange,lon=lonRange)
U = dsU['u'].sel(time=dateList,lev=pLevel,lat=latRange,lon=lonRange)
V = dsV['v'].sel(time=dateList,lev=pLevel,lat=latRange,lon=lonRange)
T
<xarray.DataArray 't' (time: 1, lat: 31, lon: 61)> array([[[264.6 , 264.80002, ..., 276.2 , 276.2 ], [263.9 , 264.30002, ..., 276. , 276.1 ], ..., [250.5 , 250.2 , ..., 266.80002, 266.7 ], [249.90001, 249.7 , ..., 266.4 , 266.4 ]]], dtype=float32) Coordinates: * time (time) datetime64[ns] 2007-02-14T12:00:00 lev float32 850.0 * lat (lat) float32 35.0 35.5 36.0 36.5 37.0 ... 48.0 48.5 49.0 49.5 50.0 * lon (lon) float32 -90.0 -89.5 -89.0 -88.5 ... -61.5 -61.0 -60.5 -60.0 Attributes: level_type: Isobaric (hPa) units: K long_name: temperature
In order to calculate many meteorologically-relevant quantities that depend on distances between gridpoints, we need horizontal distance between gridpoints in meters. MetPy can infer this from datasets such as our CFSR that are lat-lon based, but we need to explicitly assign a coordinate reference system first.¶
3) Calculate and visualize diagnostic quantities.¶
T
<xarray.DataArray 't' (time: 1, lat: 31, lon: 61)> array([[[264.6 , 264.80002, ..., 276.2 , 276.2 ], [263.9 , 264.30002, ..., 276. , 276.1 ], ..., [250.5 , 250.2 , ..., 266.80002, 266.7 ], [249.90001, 249.7 , ..., 266.4 , 266.4 ]]], dtype=float32) Coordinates: * time (time) datetime64[ns] 2007-02-14T12:00:00 lev float32 850.0 * lat (lat) float32 35.0 35.5 36.0 36.5 37.0 ... 48.0 48.5 49.0 49.5 50.0 * lon (lon) float32 -90.0 -89.5 -89.0 -88.5 ... -61.5 -61.0 -60.5 -60.0 Attributes: level_type: Isobaric (hPa) units: K long_name: temperature
crsCFSR = {'grid_mapping_name': 'latitude_longitude', 'earth_radius': 6371229.0}
T = T.metpy.assign_crs(crsCFSR)
U = U.metpy.assign_crs(crsCFSR)
V = V.metpy.assign_crs(crsCFSR)
T.metpy.assign_crs(crsCFSR)
<xarray.DataArray 't' (time: 1, lat: 31, lon: 61)> array([[[264.6 , 264.80002, ..., 276.2 , 276.2 ], [263.9 , 264.30002, ..., 276. , 276.1 ], ..., [250.5 , 250.2 , ..., 266.80002, 266.7 ], [249.90001, 249.7 , ..., 266.4 , 266.4 ]]], dtype=float32) Coordinates: * time (time) datetime64[ns] 2007-02-14T12:00:00 lev float32 850.0 * lat (lat) float32 35.0 35.5 36.0 36.5 37.0 ... 48.5 49.0 49.5 50.0 * lon (lon) float32 -90.0 -89.5 -89.0 -88.5 ... -61.5 -61.0 -60.5 -60.0 metpy_crs object Projection: latitude_longitude Attributes: level_type: Isobaric (hPa) units: K long_name: temperature
Define our subsetted coordinate arrays of lat and lon. Pull them from any of the DataArrays. We’ll need to pass these into the contouring functions later on.
lats = T.lat
lons = T.lon
First, let’s just plot contour lines of temperature and wind barbs.¶
Unit conversions¶
While we will simply redefine our temperature object so it is in Celsius, let’s create two new objects for U and V in knots, since we will want to preserve the original units (m/s) when it comes time to calculate temperature advection.
T = T.metpy.convert_units('degC')
UKts = U.metpy.convert_units('kts')
VKts = V.metpy.convert_units('kts')
Create an array of values for the temperature contours: since we are using contour lines, simply define a range large enough to encompass the expected values, and set a contour interval.
T.min().values, T.max().values
(array(-24.749985, dtype=float32), array(10.25, dtype=float32))
cint = 2
minT, maxT = (-30,22)
TContours = np.arange(minT, maxT, cint)
Make the map¶
constrainLat, constrainLon = (0.5, 4.0)
proj_map = ccrs.LambertConformal(central_longitude=cLon, central_latitude=cLat)
proj_data = ccrs.PlateCarree() # Our data is lat-lon; thus its native projection is Plate Carree.
res = '50m'
Although there is just a single time in our time range, we’ll still employ a loop here in case we wanted to include multiple times later on.
for time in dateList:
print("Processing", time)
# Create the time strings, for the figure title as well as for the file name.
timeStr = dt.strftime(time,format="%Y-%m-%d %H%M UTC")
timeStrFile = dt.strftime(time,format="%Y%m%d%H")
tl1 = str('CFSR, Valid at: '+ timeStr)
tl2 = levStr + " hPa temperature ($^\circ$C) and winds (kts)"
title_line = (tl1 + '\n' + tl2 + '\n')
fig = plt.figure(figsize=(21,15)) # Increase size to adjust for the constrained lats/lons
ax = plt.subplot(1,1,1,projection=proj_map)
ax.set_extent ([lonW+constrainLon,lonE-constrainLon,latS+constrainLat,latN-constrainLat])
ax.add_feature(cfeature.COASTLINE.with_scale(res))
ax.add_feature(cfeature.STATES.with_scale(res),edgecolor='brown')
# Need to use Xarray's sel method here to specify the current time for any DataArray you are plotting.
# 1. Contour lines of temperature
cT = ax.contour(lons, lats, T.sel(time=time), levels=TContours, colors='orange', linewidths=3, transform=proj_data)
ax.clabel(cT, inline=1, fontsize=12, fmt='%.0f')
# 4. wind barbs
# Plotting wind barbs uses the ax.barbs method. Here, you can't pass in the DataArray directly; you can only pass in the array's values.
# Also need to sample (skip) a selected # of points to keep the plot readable.
# Remember to use Xarray's sel method here as well to specify the current time.
skip = 2
ax.barbs(lons[::skip],lats[::skip],UKts.sel(time=time)[::skip,::skip].values, VKts.sel(time=time)[::skip,::skip].values, color='purple',zorder=2,transform=proj_data)
title = plt.title(title_line,fontsize=16)
Processing 2007-02-14 12:00:00
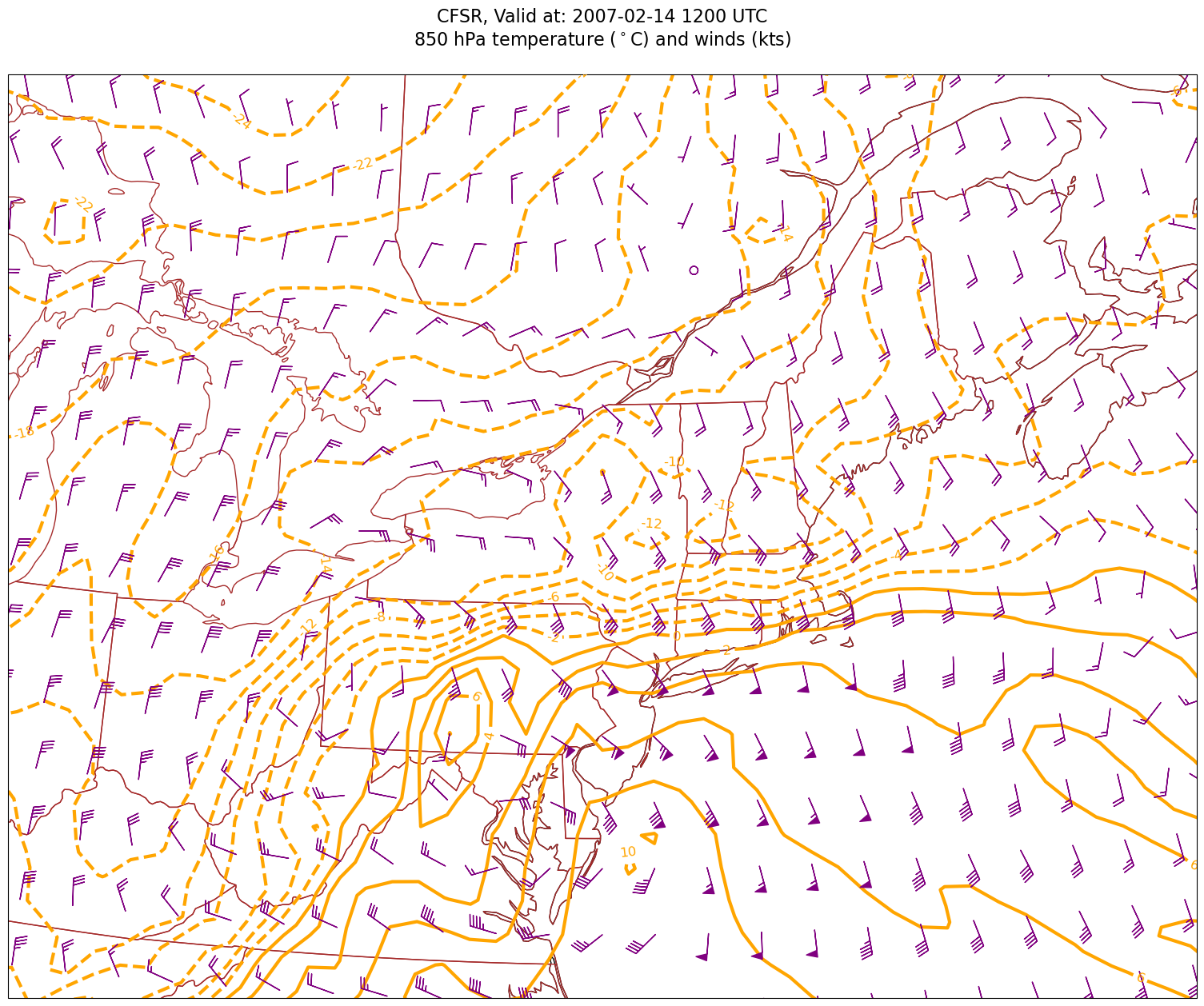
Look at the map and you should easily be able to identify the areas of prominent warm and cold advection. Instead of qualitatively assessing a diagnostic quantity such as temperature advection, how about we quantify it? For that, we will use MetPy’s diagnostic library, which we have imported as mpcalc
.¶
MetPy Diagnostics¶
Let’s explore the advection
diagnostic: https://unidata.github.io/MetPy/latest/api/generated/metpy.calc.advection.html¶
In order to calculate horizontal tempareture advection, we need a scalar quantity (temperature, the “thing” being advected), and a vector field (wind, the “thing” doing the advecting).¶
For MetPy’s advection function, we pass in the scalar, followed by the vector … in this case, T followed by U and V.
# Calculate temperature advection by the horizontal wind:
tAdv = mpcalc.advection(T, U, V)
/knight/anaconda_aug22/envs/aug22_env/lib/python3.10/site-packages/metpy/xarray.py:1473: UserWarning: Vertical dimension number not found. Defaulting to (..., Z, Y, X) order.
warnings.warn(
Let’s look at the output DataArray
, containing the calculated values (and units) of temperature advection. Note how these values scale … on the order of 10**-5.
tAdv
<xarray.DataArray (time: 1, lat: 31, lon: 61)> <Quantity([[[-3.32239952e-04 -1.17486361e-04 -1.79719079e-04 ... 1.36805743e-04 8.86109701e-05 3.30358230e-05] [-5.80205203e-05 3.02556066e-05 -9.09141394e-05 ... 2.30428254e-05 -1.49712672e-05 -3.19765032e-05] [ 9.24928691e-05 7.94499917e-05 -8.19642744e-06 ... -5.11116791e-05 -4.35406925e-05 -2.13607897e-05] ... [-7.62280949e-05 -1.61135810e-04 -1.57744313e-04 ... -3.77502292e-05 -2.65168282e-05 -2.54256012e-05] [-6.37189651e-05 -1.13801928e-04 -1.53448797e-04 ... -5.73476184e-05 -5.07743885e-05 -3.06682474e-05] [-1.03128098e-04 -6.00530185e-05 -1.21533033e-04 ... -6.24078314e-05 -7.93525520e-05 -8.38959291e-05]]], 'kelvin / second')> Coordinates: * time (time) datetime64[ns] 2007-02-14T12:00:00 lev float32 850.0 * lat (lat) float32 35.0 35.5 36.0 36.5 37.0 ... 48.5 49.0 49.5 50.0 * lon (lon) float32 -90.0 -89.5 -89.0 -88.5 ... -61.5 -61.0 -60.5 -60.0 metpy_crs object Projection: latitude_longitude
Let’s get a quick visualization, using Xarray’s built-in interface to Matplotlib.
tAdv.plot(figsize=(15,10),cmap='coolwarm')
<matplotlib.collections.QuadMesh at 0x14b33d7ea740>
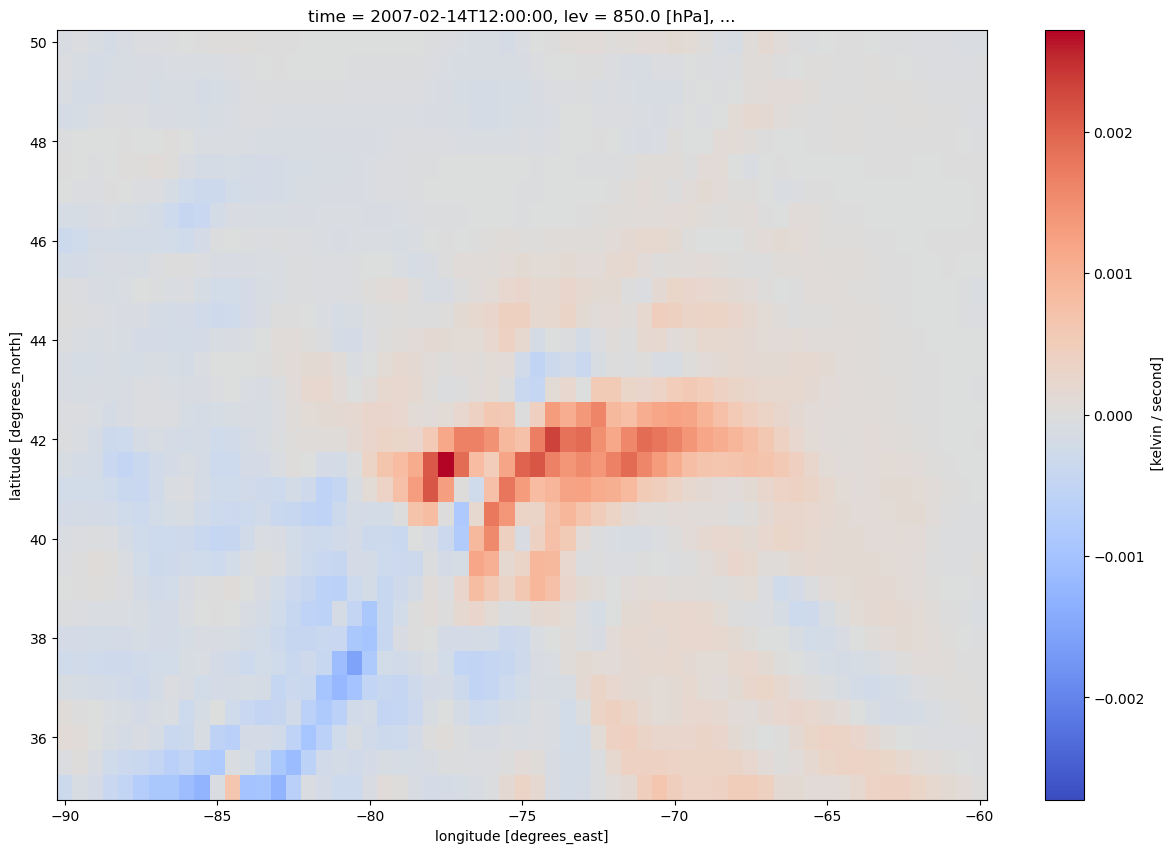
We have three strategies to make the resulting map look nicer:¶
Scale up the values by an appropriate power of ten
Focus on the more extreme values of temperature advection: thus, do not contour values that are close to zero.
Smooth the values of temperature advection … especially important in datasets with a high degree of horizontal resolution.
Let’s take care of the first two strategies, and then assess the need for smoothing.
Scale these values up by 1e5 (or 1 * 10**5, or 100,000) and find the min/max values. Use these to inform the setting of the contour fill intervals.¶
scale = 1e5
minTAdv = (tAdv*scale).min().values
maxTAdv = (tAdv*scale).max().values
print (minTAdv, maxTAdv)
-156.30034272620907 272.3350283440195
Usually, we wish to avoid plotting the “zero” contour line for diagnostic quantities such as divergence, advection, and frontogenesis. Thus, create two lists of values … one for negative and one for positive.¶
advInc = 50
negAdvContours = np.arange (-300, 0, advInc)
posAdvContours = np.arange (50, 350, advInc)
negAdvContours
array([-300, -250, -200, -150, -100, -50])
Now, let’s plot temperature advection on the map.¶
for time in dateList:
print("Processing", time)
# Create the time strings, for the figure title as well as for the file name.
timeStr = dt.strftime(time,format="%Y-%m-%d %H%M UTC")
timeStrFile = dt.strftime(time,format="%Y%m%d%H")
tl1 = str('CFSR, Valid at: '+ timeStr)
tl2 = levStr + " hPa temperature advection ($^\circ$C x 10**5 / s)"
title_line = (tl1 + '\n' + tl2 + '\n')
fig = plt.figure(figsize=(21,15)) # Increase size to adjust for the constrained lats/lons
ax = plt.subplot(1,1,1,projection=proj_map)
ax.set_extent ([lonW+constrainLon,lonE-constrainLon,latS+constrainLat,latN-constrainLat])
ax.add_feature(cfeature.COASTLINE.with_scale(res))
ax.add_feature(cfeature.STATES.with_scale(res),edgecolor='brown')
# Need to use Xarray's sel method here to specify the current time for any DataArray you are plotting.
# 1a. Contour lines of warm (positive temperature) advection.
# Don't forget to multiply by the scaling factor!
cPosTAdv = ax.contour(lons, lats, tAdv.sel(time=time)*scale, levels=posAdvContours, colors='red', linewidths=3, transform=proj_data)
ax.clabel(cPosTAdv, inline=1, fontsize=12, fmt='%.0f')
# 1b. Contour lines of cold (negative temperature) advection
cNegTAdv = ax.contour(lons, lats, tAdv.sel(time=time)*scale, levels=negAdvContours, colors='blue', linewidths=3, transform=proj_data)
ax.clabel(cNegTAdv, inline=1, fontsize=12, fmt='%.0f')
title = plt.title(title_line,fontsize=16)
#Generate a string for the file name and save the graphic to your current directory.
fileName = timeStrFile + '_CFSR_' + levStr + '_TAdv.png'
fig.savefig(fileName)
Processing 2007-02-14 12:00:00
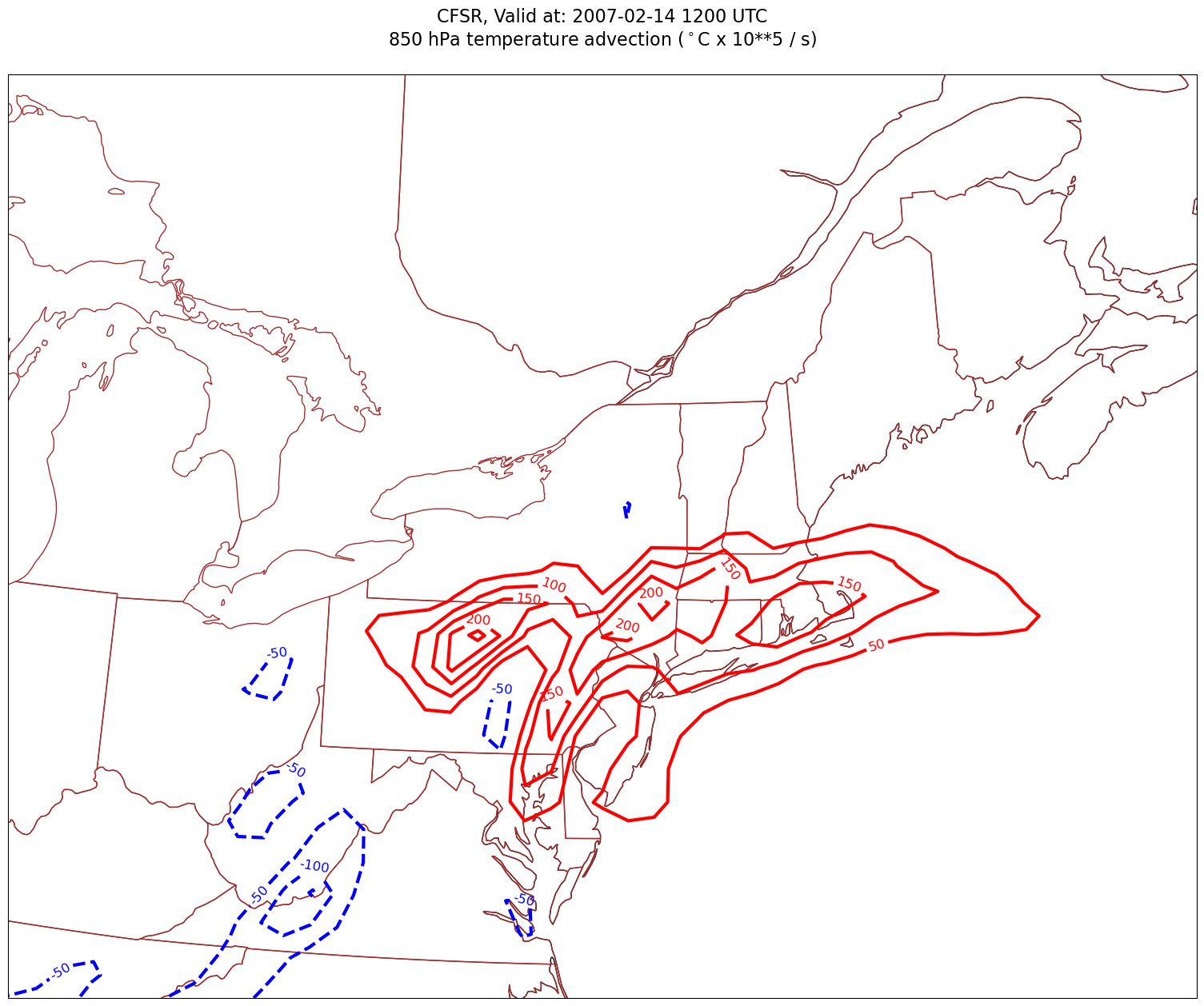
4. Smooth the diagnostic field.¶
This is not a terribly “noisy” field. But let’s demonstrate the technique of employing a smoothing function. We will use a Gaussian filter, from the SciPy library, which, along with NumPy and Pandas, is one of the core packages in Python’s scientific software ecosystem.
MetPy now includes the Gaussian smoother as one of its diagnostics.
sigma = 8.0 # this depends on how noisy your data is, adjust as necessary
tAdvSmooth = mpcalc.smooth_gaussian(tAdv, sigma)
As we did before with the unsmoothed values, let’s look at the resulting DataArray
and its extrema.
tAdvSmooth
<xarray.DataArray (time: 1, lat: 31, lon: 61)> <Quantity([[[-1.38886924e-04 -1.42960509e-04 -1.91862232e-04 ... 8.50491727e-05 4.78767630e-05 2.71095779e-05] [-7.66734512e-05 -8.94489884e-05 -1.40727649e-04 ... 4.46418566e-05 2.15498618e-05 9.24863080e-06] [-2.58699961e-05 -4.54471033e-05 -9.33043145e-05 ... 2.68579056e-06 -9.10384659e-07 -2.33512111e-06] ... [-1.02154143e-04 -1.05318859e-04 -1.03849236e-04 ... -2.69051135e-05 -3.28996428e-05 -3.59743427e-05] [-1.00189424e-04 -1.09018682e-04 -1.17056533e-04 ... -4.32947544e-05 -4.74230042e-05 -4.87006125e-05] [-9.56115030e-05 -1.05697051e-04 -1.18446813e-04 ... -5.39039147e-05 -5.83224792e-05 -5.99163454e-05]]], 'kelvin / second')> Coordinates: * time (time) datetime64[ns] 2007-02-14T12:00:00 lev float32 850.0 * lat (lat) float32 35.0 35.5 36.0 36.5 37.0 ... 48.5 49.0 49.5 50.0 * lon (lon) float32 -90.0 -89.5 -89.0 -88.5 ... -61.5 -61.0 -60.5 -60.0 metpy_crs object Projection: latitude_longitude
scale = 1e5
minTAdv = (tAdvSmooth * scale).min().values
maxTAdv = (tAdvSmooth * scale).max().values
print (minTAdv, maxTAdv)
-76.07511957712059 136.9952162002647
Not surprisingly, the magnitude of the extrema decreased.
Plot the smoothed advection field.
for time in dateList:
print("Processing", time)
# Create the time strings, for the figure title as well as for the file name.
timeStr = dt.strftime(time,format="%Y-%m-%d %H%M UTC")
timeStrFile = dt.strftime(time,format="%Y%m%d%H")
tl1 = str('CFSR, Valid at: '+ timeStr)
tl2 = levStr + " hPa temperature advection ($^\circ$C x 10**5 / s), smoothed"
title_line = (tl1 + '\n' + tl2 + '\n')
fig = plt.figure(figsize=(21,15)) # Increase size to adjust for the constrained lats/lons
ax = plt.subplot(1,1,1,projection=proj_map)
ax.set_extent ([lonW+constrainLon,lonE-constrainLon,latS+constrainLat,latN-constrainLat])
ax.add_feature(cfeature.COASTLINE.with_scale(res))
ax.add_feature(cfeature.STATES.with_scale(res),edgecolor='brown')
# Need to use Xarray's sel method here to specify the current time for any DataArray you are plotting.
# 1a. Contour lines of warm (positive temperature) advection.
# Don't forget to multiply by the scaling factor!
cPosTAdv = ax.contour(lons, lats, tAdvSmooth.sel(time=time)*scale, levels=posAdvContours, colors='red', linewidths=3, transform=proj_data)
ax.clabel(cPosTAdv, inline=1, fontsize=12, fmt='%.0f')
# 1b. Contour lines of cold (negative temperature) advection
cNegTAdv = ax.contour(lons, lats, tAdvSmooth.sel(time=time)*scale, levels=negAdvContours, colors='blue', linewidths=3, transform=proj_data)
ax.clabel(cNegTAdv, inline=1, fontsize=12, fmt='%.0f')
title = plt.title(title_line,fontsize=16)
#Generate a string for the file name and save the graphic to your current directory.
fileName = timeStrFile + '_CFSR_' + levStr + '_TAdvSmth.png'
fig.savefig(fileName)
Processing 2007-02-14 12:00:00
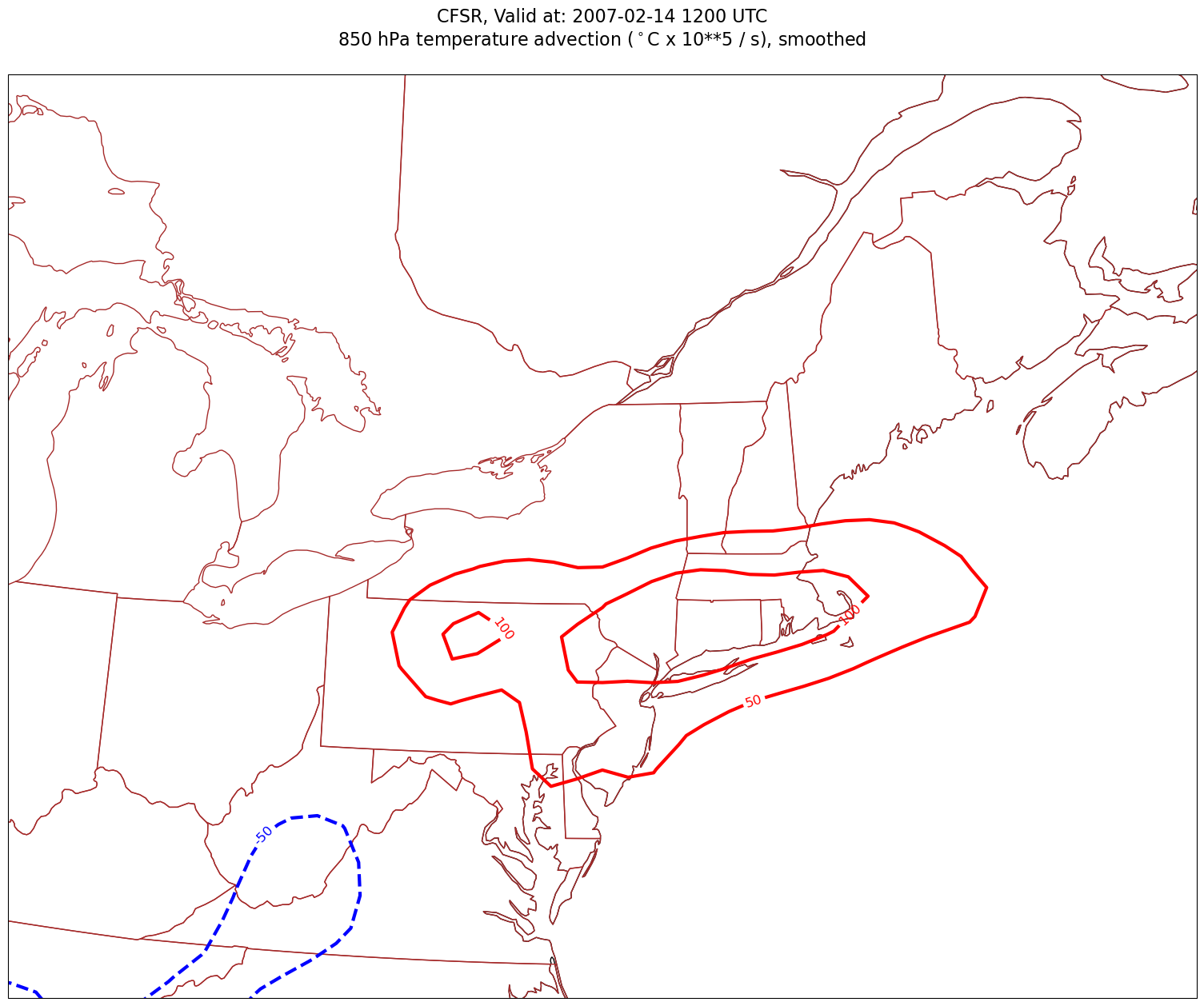